์ปค๋งจ๋ ํจํด ์ ์ ) ์ปค๋งจ๋ ํจํด์ ์ด์ฉํ๋ฉด ์๊ตฌ์ฌํญ์ ๊ฐ์ฒด๋ก ์บก์ํ ํ ์ ์์ผ๋ฉฐ, ๋งค๊ฐ๋ณ์๋ฅผ ์จ์ ์ฌ๋ฌ๊ฐ์ง ๋ค๋ฅธ ์๊ตฌ ์ฌํญ์ ์ง์ด ๋ฃ์์ ์๋ค. ๋ํ, ์์ฒญ ๋ด์ญ์ ํ์ ์ ์ฅํ๊ฑฐ๋ ๋ก๊ทธ๋ฅผ ๊ธฐ๋กํ์ฌ, ์์ ์ทจ์ ๊ธฐ๋ฅ๋ ๋ฃ์์ ์๋ค.
์ปค๋งจํธ ํจํด์ ์ผ๋ จ์ ํ๋์ ํน์ ๋ฆฌ์๋ฒ ํ๊ณ ์ฐ๊ฒฐ ์ํด์ผ๋ก์จ ์๊ตฌ ์ฌํญ์ ์บก์ํ ์ํจ๋ค.
์์ฒญ์ ํ๋ ๊ฐ์ฒด์ ๊ทธ ์์ฒญ์ ์ํํ๋ ๊ฐ์ฒด๋ฅผ ๋ถ๋ฆฌ์ํค๊ณ ์ถ๋ค๋ฉด ์ปค๋งจ๋ ํจํด์ ์ฌ์ฉํ๋ฉด ๋๋ค.
์ปค๋งจ๋ ํจํด : ํด๋์ค ๋ค์ด์ด๊ทธ๋จ
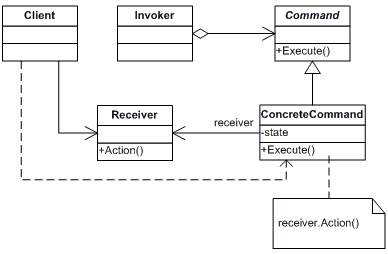
Remote Control ์์
public class LightOnCommand implements Command {
Light light;
public LightOnCommand(Light light) {
this.light = light;
}
public void execute() {
light.on();
}
}
public class RemoteControl {
Command[] onCommands; // on ๋ฒํผ๋ค ๋ชจ์๋๋ ๋ฐฐ์ด
Command[] offCommands; // off ๋ฒํผ๋ค ๋ชจ์๋๋ ๋ฐฐ์ด
public RemoteControl() {
onCommands = new Command[7];
offCommands = new Command[7];
Command noCommand = new NoCommand();
for (int i = 0; i < 7; i++) {
onCommands[i] = noCommand; // ์ผ๋จ ์ด๊ธฐํ๋ฅผ ์ปค๋งจ๋๊ฐ ์๋ noCommand ๊ฐ์ฒด ๋ฃ์.
offCommands[i] = noCommand; // ์ผ๋จ ์ด๊ธฐํ๋ฅผ ์ปค๋งจ๋๊ฐ ์๋ noCommand ๊ฐ์ฒด ๋ฃ์.
}
}
public void setCommand(int slot, Command onCommand, Command offCommand) {
onCommands[slot] = onCommand;
offCommands[slot] = offCommand;
}
public void onButtonWasPushed(int slot) { // slot์ ํด๋นํ๋ ๊ฐ์ฒด on ์คํ(execute)
onCommands[slot].execute();
}
public void offButtonWasPushed(int slot) { // slot์ ํด๋นํ๋ ๊ฐ์ฒด off ์คํ(execute)
offCommands[slot].execute();
}
public String toString() {
StringBuffer stringBuff = new StringBuffer();
stringBuff.append("\n------ Remote Control -------\n");
for (int i = 0; i < onCommands.length; i++) {
stringBuff.append("[slot " + i + "] " + onCommands[i].getClass().getName()
+ " " + offCommands[i].getClass().getName() + "\n");
}
return stringBuff.toString();
}
}
public class RemoteLoader {
public static void main(String[] args) {
RemoteControl remoteControl = new RemoteControl(); //๋ฆฌ๋ชจ์ปจ ๊ฐ์ฒด ์์ฑ
//living room์ ์๋ Light ๊ฐ์ฒด ์์ฑ
Light livingRoomLight = new Light("Living Room");
//on,off์ปค๋งจ๋์ Light ์์น๋ฅผ ๋๊ฒจ์ค.
LightOnCommand livingRoomLightOn =
new LightOnCommand(livingRoomLight);
LightOffCommand livingRoomLightOff =
new LightOffCommand(livingRoomLight);
//on,off command ๋ฑ๋ก
remoteControl.setCommand(0, livingRoomLightOn, livingRoomLightOff);
System.out.println(remoteControl);
remoteControl.onButtonWasPushed(0);
remoteControl.offButtonWasPushed(0);
}
}
์ถ๋ ฅ ) [slot 0] package.LightOnCommand package.LightOffCommand
( noCommand ..์๋ต )
Living Room light is on
Living Room light is off
๊ฐ๋จํ ๋ฆฌ๋ชจ์ปจ : ํด๋์ค ๋ค์ด์ด๊ทธ๋จ
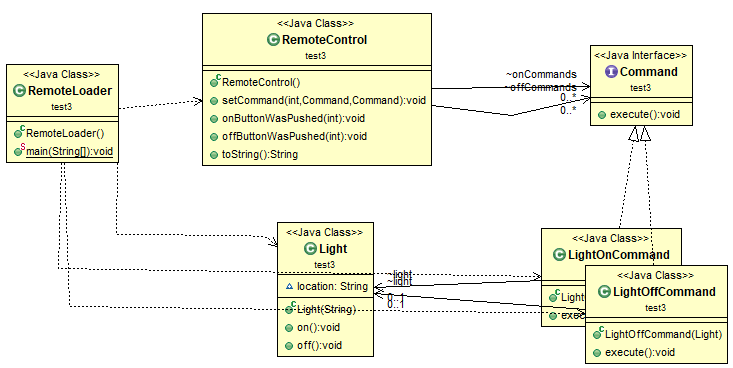
๋ฆฌ๋ชจ์ปจ์ Undo() ๊ธฐ๋ฅ ์ถ๊ฐํ๊ธฐ.
public interface Command{
public void execute();
public void undo(); // <<-- undo๋ง ์ถ๊ฐํด์ฃผ๋ฉด ๋จ!
}
//LightOnCommand
public void execute(){
light.on();
}
public void undo(){
light.off(); // on -> off / off -> on
}
public class RemoteControlWithUndo {
Command[] onCommands;
Command[] offCommands;
Command undoCommand; // new!
public RemoteControlWithUndo() {
onCommands = new Command[7];
offCommands = new Command[7];
Command noCommand = new NoCommand();
for(int i=0;i<7;i++) {
onCommands[i] = noCommand;
offCommands[i] = noCommand;
}
undoCommand = noCommand; // new!
}
public void setCommand(int slot, Command onCommand, Command offCommand) {
onCommands[slot] = onCommand;
offCommands[slot] = offCommand;
}
public void onButtonWasPushed(int slot) {
onCommands[slot].execute();
undoCommand = onCommands[slot]; // new!
}
public void offButtonWasPushed(int slot) {
offCommands[slot].execute();
undoCommand = offCommands[slot]; // new!
}
public void undoButtonWasPushed() { // new!
undoCommand.undo();
}
//toString() ์๋ต.
}
party mode
public class MacroCommand implements Command{
Command[] commands;
public MacroCommand( Command[] commands){
this.commands = commands;
}
public void execute(){
for(int i=0; i< commands.length; i++){
commands[i].execute();
}
}
//Macro ๋ฑ๋ก.
Command[] partyOn = {lightOn, stereoOn, tvOn, hottubOn};
Command[] partyOff = {lightOff, stereoOff, tvOff, hottubOff};
MacroCommand partyOnMacro = new MacroCommand(partyOn);
MacroCommand partyOffMacro = new MacroCommand(partyOff);
์ปค๋งจ๋ ํจํด ์ ์ ) ์ปค๋งจ๋ ํจํด์ ์ด์ฉํ๋ฉด ์๊ตฌ์ฌํญ์ ๊ฐ์ฒด๋ก ์บก์ํ ํ ์ ์์ผ๋ฉฐ, ๋งค๊ฐ๋ณ์๋ฅผ ์จ์ ์ฌ๋ฌ๊ฐ์ง ๋ค๋ฅธ ์๊ตฌ ์ฌํญ์ ์ง์ด ๋ฃ์์ ์๋ค. ๋ํ, ์์ฒญ ๋ด์ญ์ ํ์ ์ ์ฅํ๊ฑฐ๋ ๋ก๊ทธ๋ฅผ ๊ธฐ๋กํ์ฌ, ์์ ์ทจ์ ๊ธฐ๋ฅ๋ ๋ฃ์์ ์๋ค.
์ปค๋งจํธ ํจํด์ ์ผ๋ จ์ ํ๋์ ํน์ ๋ฆฌ์๋ฒ ํ๊ณ ์ฐ๊ฒฐ ์ํด์ผ๋ก์จ ์๊ตฌ ์ฌํญ์ ์บก์ํ ์ํจ๋ค.
์์ฒญ์ ํ๋ ๊ฐ์ฒด์ ๊ทธ ์์ฒญ์ ์ํํ๋ ๊ฐ์ฒด๋ฅผ ๋ถ๋ฆฌ์ํค๊ณ ์ถ๋ค๋ฉด ์ปค๋งจ๋ ํจํด์ ์ฌ์ฉํ๋ฉด ๋๋ค.
์ปค๋งจ๋ ํจํด : ํด๋์ค ๋ค์ด์ด๊ทธ๋จ
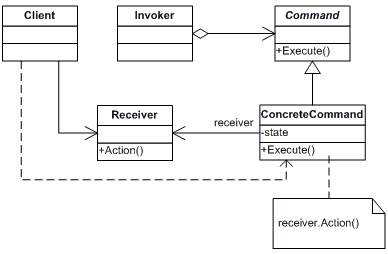
Remote Control ์์
public class LightOnCommand implements Command {
Light light;
public LightOnCommand(Light light) {
this.light = light;
}
public void execute() {
light.on();
}
}
public class RemoteControl {
Command[] onCommands; // on ๋ฒํผ๋ค ๋ชจ์๋๋ ๋ฐฐ์ด
Command[] offCommands; // off ๋ฒํผ๋ค ๋ชจ์๋๋ ๋ฐฐ์ด
public RemoteControl() {
onCommands = new Command[7];
offCommands = new Command[7];
Command noCommand = new NoCommand();
for (int i = 0; i < 7; i++) {
onCommands[i] = noCommand; // ์ผ๋จ ์ด๊ธฐํ๋ฅผ ์ปค๋งจ๋๊ฐ ์๋ noCommand ๊ฐ์ฒด ๋ฃ์.
offCommands[i] = noCommand; // ์ผ๋จ ์ด๊ธฐํ๋ฅผ ์ปค๋งจ๋๊ฐ ์๋ noCommand ๊ฐ์ฒด ๋ฃ์.
}
}
public void setCommand(int slot, Command onCommand, Command offCommand) {
onCommands[slot] = onCommand;
offCommands[slot] = offCommand;
}
public void onButtonWasPushed(int slot) { // slot์ ํด๋นํ๋ ๊ฐ์ฒด on ์คํ(execute)
onCommands[slot].execute();
}
public void offButtonWasPushed(int slot) { // slot์ ํด๋นํ๋ ๊ฐ์ฒด off ์คํ(execute)
offCommands[slot].execute();
}
public String toString() {
StringBuffer stringBuff = new StringBuffer();
stringBuff.append("\n------ Remote Control -------\n");
for (int i = 0; i < onCommands.length; i++) {
stringBuff.append("[slot " + i + "] " + onCommands[i].getClass().getName()
+ " " + offCommands[i].getClass().getName() + "\n");
}
return stringBuff.toString();
}
}
public class RemoteLoader {
public static void main(String[] args) {
RemoteControl remoteControl = new RemoteControl(); //๋ฆฌ๋ชจ์ปจ ๊ฐ์ฒด ์์ฑ
//living room์ ์๋ Light ๊ฐ์ฒด ์์ฑ
Light livingRoomLight = new Light("Living Room");
//on,off์ปค๋งจ๋์ Light ์์น๋ฅผ ๋๊ฒจ์ค.
LightOnCommand livingRoomLightOn =
new LightOnCommand(livingRoomLight);
LightOffCommand livingRoomLightOff =
new LightOffCommand(livingRoomLight);
//on,off command ๋ฑ๋ก
remoteControl.setCommand(0, livingRoomLightOn, livingRoomLightOff);
System.out.println(remoteControl);
remoteControl.onButtonWasPushed(0);
remoteControl.offButtonWasPushed(0);
}
}
์ถ๋ ฅ ) [slot 0] package.LightOnCommand package.LightOffCommand
( noCommand ..์๋ต )
Living Room light is on
Living Room light is off
๊ฐ๋จํ ๋ฆฌ๋ชจ์ปจ : ํด๋์ค ๋ค์ด์ด๊ทธ๋จ
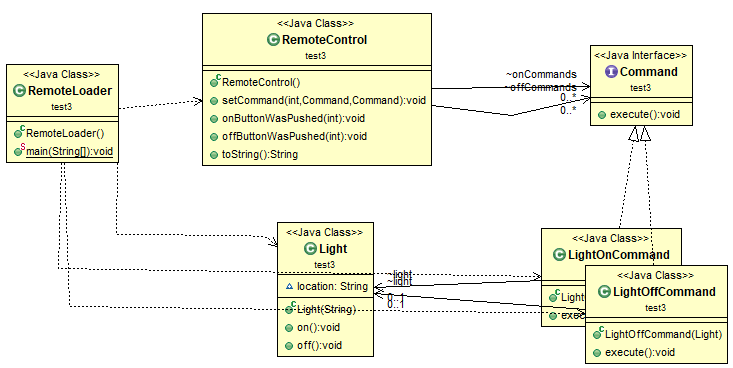
๋ฆฌ๋ชจ์ปจ์ Undo() ๊ธฐ๋ฅ ์ถ๊ฐํ๊ธฐ.
public interface Command{
public void execute();
public void undo(); // <<-- undo๋ง ์ถ๊ฐํด์ฃผ๋ฉด ๋จ!
}
//LightOnCommand
public void execute(){
light.on();
}
public void undo(){
light.off(); // on -> off / off -> on
}
public class RemoteControlWithUndo {
Command[] onCommands;
Command[] offCommands;
Command undoCommand; // new!
public RemoteControlWithUndo() {
onCommands = new Command[7];
offCommands = new Command[7];
Command noCommand = new NoCommand();
for(int i=0;i<7;i++) {
onCommands[i] = noCommand;
offCommands[i] = noCommand;
}
undoCommand = noCommand; // new!
}
public void setCommand(int slot, Command onCommand, Command offCommand) {
onCommands[slot] = onCommand;
offCommands[slot] = offCommand;
}
public void onButtonWasPushed(int slot) {
onCommands[slot].execute();
undoCommand = onCommands[slot]; // new!
}
public void offButtonWasPushed(int slot) {
offCommands[slot].execute();
undoCommand = offCommands[slot]; // new!
}
public void undoButtonWasPushed() { // new!
undoCommand.undo();
}
//toString() ์๋ต.
}
party mode
public class MacroCommand implements Command{
Command[] commands;
public MacroCommand( Command[] commands){
this.commands = commands;
}
public void execute(){
for(int i=0; i< commands.length; i++){
commands[i].execute();
}
}
//Macro ๋ฑ๋ก.
Command[] partyOn = {lightOn, stereoOn, tvOn, hottubOn};
Command[] partyOff = {lightOff, stereoOff, tvOff, hottubOff};
MacroCommand partyOnMacro = new MacroCommand(partyOn);
MacroCommand partyOffMacro = new MacroCommand(partyOff);